[Laravel] Adding custom data to eloquent result
- 2021年6月07日
- 技術情報
Sometimes, we want to add more custom data to our eloquent result before passing to blade or doing further logic improvements. Today we will be focusing how to add custom data to our eloquent result.
I assume you already know laravel framework in this article as we will not covering from scratch of laravel framework. First let’s make a posts table. Here is the migration file.
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('description');
$table->timestamps();
});
In our post table, we will have these columns in above. And we gonna add a data record. You can add manually using database clients or you can of course make a seeder class to add dummy data like this
DB::table('posts')->insert([
'name' => Str::random(10),
'description' => Str::random(100),
'created_at' => date('Y-m-d H:i:s'),
]);
Lets go to our controller and select the data with all method and print the result with dd function.
$data = Post::all();
dd($data);
The output will be look like this.
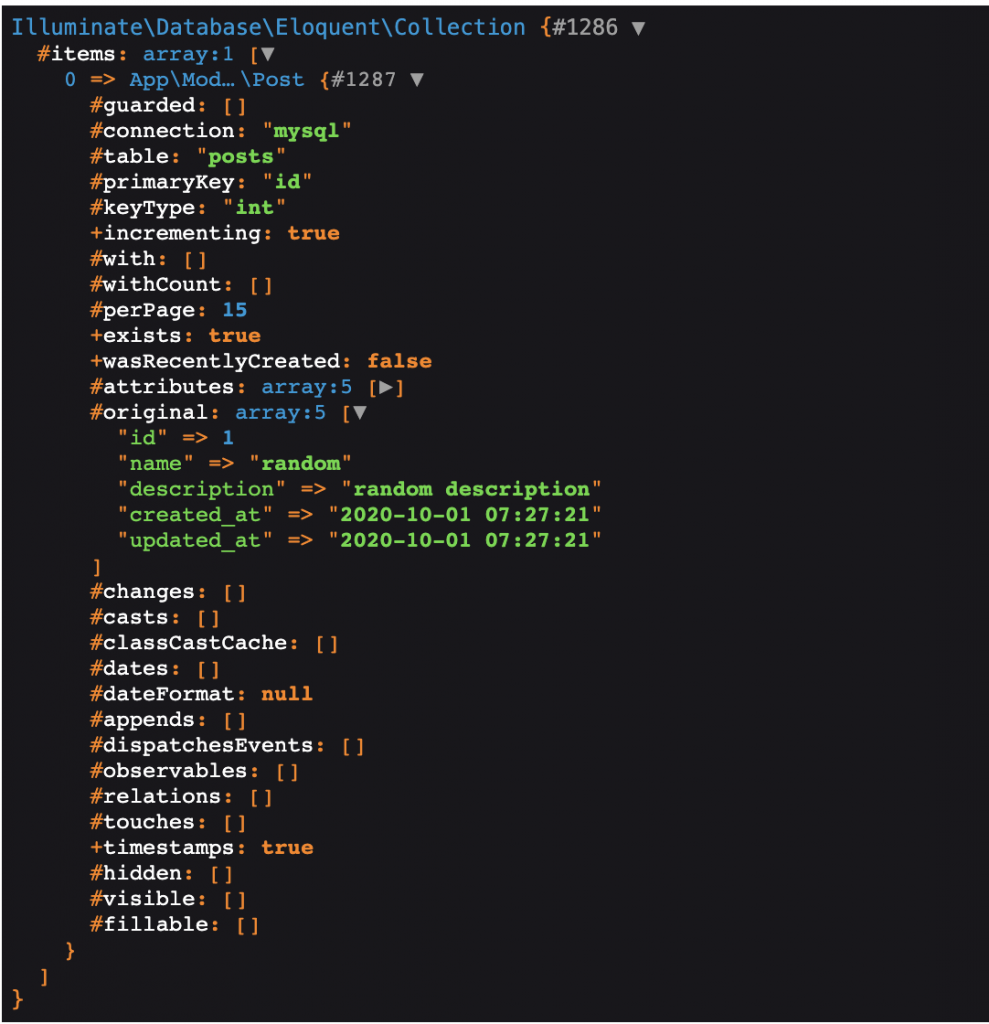
Now , let’s try to add a new column called category_id
and user_id
to that eloquent results. We can achieve this using map function.
$data = Post::all();
$data->map(function ($result) {
$result->category_id = 1;
$result->user_id = 2;
return $result;
});
dd($data);
And the result will be look like this. Please remember there are two arrays called attributes
which is the one we customized and added the data. And there is also original
array which contains only original data from eloquent.

Anyhow, we can access our created data using map like always. For further more to customize the eloquent results , please check out here.
dd($data[0]->category_id.','.$data[0]->user_id);
//result -> 1,2
If you need to loop through many data, you can use loop functions but in this case I just added a 0 index to get data easily.
By Yuuma
yuuma at 2021年06月07日 11:10:35