技術情報
- 2023年04月25日
- 技術情報
Creating client and server sockets using Python
Today, we will explore how to create client and server sockets using Python, along with an example code.
Client and server sockets are a fundamental part of network communication in computer science. A socket is essentially an endpoint that enables two-way communication between two devices over a network.
Server Socket
The server socket is a program that listens for incoming connections from clients. Once a connection is established, the server creates a new socket object to handle the communication with the client. Here is a basic example of a server socket program in Python:
import socket
HOST = '127.0.0.1' # Standard loopback interface address (localhost)
PORT = 65432 # Port to listen on (non-privileged ports are > 1023)
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.bind((HOST, PORT))
s.listen()
conn, addr = s.accept()
with conn:
print('Connected by', addr)
while True:
data = conn.recv(1024)
if not data:
break
conn.sendall(data)
In the above code, we first import the socket module and define the IP address and port number for the server. Then we create a new socket object ‘s’ using the AF_INET address family and SOCK_STREAM socket type.
We then bind the socket to our defined IP address and port number, and start listening for incoming connections using the s.listen() method. Once a client connects, the s.accept() method returns a new socket object ‘conn’ representing the connection, along with the address of the client.
We then use a while loop to continuously receive data from the client using conn.recv() and send data back to the client using conn.sendall() until there is no more data to receive.
Client Socket
The client socket is a program that initiates a connection to the server socket. Here is a basic example of a client socket program in Python:
import socket
HOST = '127.0.0.1' # The server's hostname or IP address
PORT = 65432 # The port used by the server
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.connect((HOST, PORT))
s.sendall(b'Hello, world')
data = s.recv(1024)
print('Received', repr(data))
In the above code, we again import the socket module and define the IP address and port number for the server. We then create a new socket object ‘s’ using the AF_INET address family and SOCK_STREAM socket type.
We then initiate a connection to the server using s.connect() and send data to the server using s.sendall(). Finally, we receive data from the server using s.recv() and print the received data.
Conclusion
We have explored how to create client and server sockets using Python along with a basic example code. Sockets are a powerful tool for network communication and are used extensively in a wide range of applications. With the above knowledge, you can build complex network applications in Python.
This is all for now. Hope you enjoy that.
By Asahi
waithaw at 2023年04月25日 10:00:00
- 2023年04月24日
- 技術情報
Google Meet now allows pausing video streams for individual tiles
Google Meet is rolling out a new feature that lets you pause streaming video for individual tiles so you can focus on the speaker or presenter who’s talking frequently.
To turn off individual sources, tap the three-dot menu next to the person’s name in the web sidebar and click Don’t See. On mobile, there’s a new “audio only” mode that disables all streams except the presenter stream. Hopefully, Google will bring this feature to the desktop as well.
The ‘audio only’ mode is certainly useful for saving data if you’re on the go.
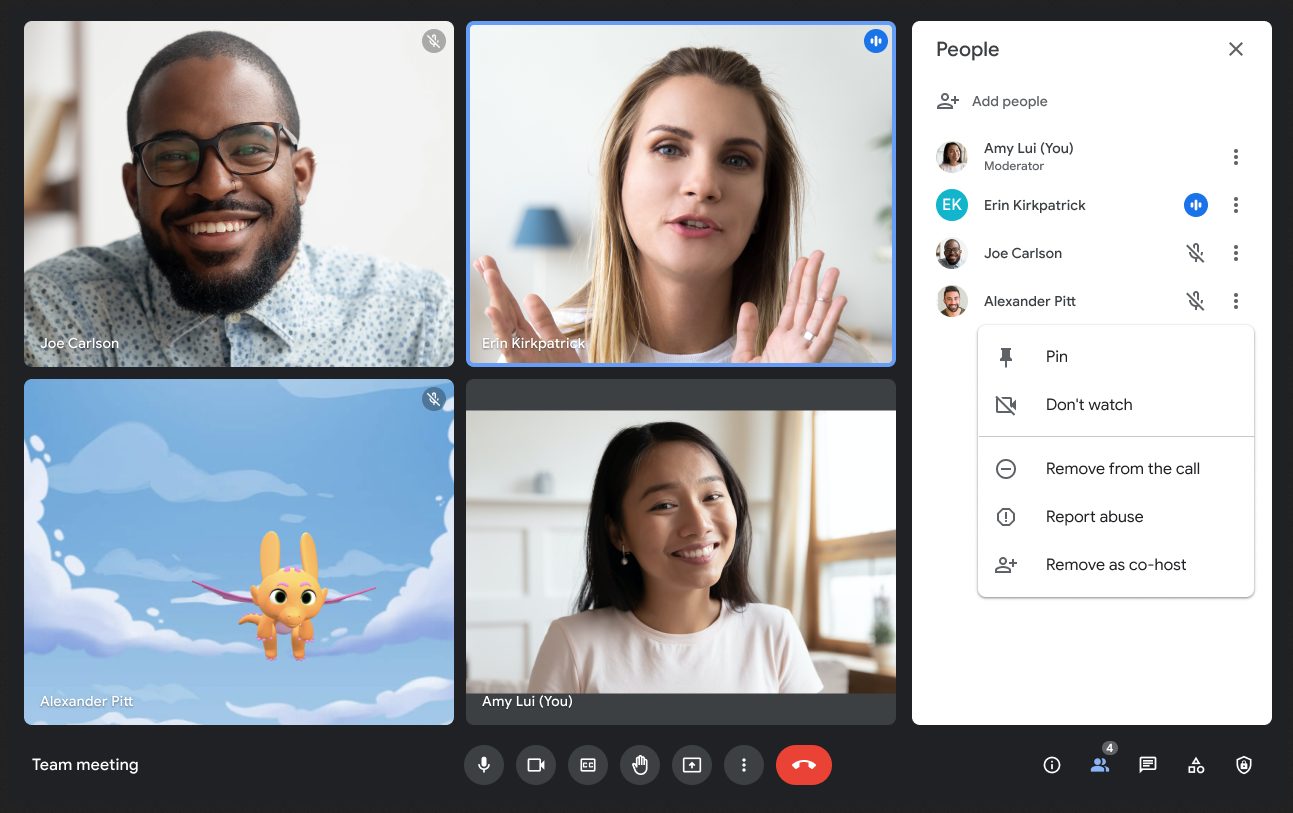
According to Google, Meet won’t notify others when you turn off the video stream. Also, the meeting experience will not change.
The company says it has already started rolling out this feature and will have it available across all locations in the coming weeks. Luckily, admins can’t disable this feature for individuals, so it’s always available to users.
Yuuma
yuuma at 2023年04月24日 10:00:00
- 2023年04月21日
- 技術情報
動画の再生・一時停止をflutterで行うためのTips
1.video_playerのDependencyを追加する。
2.アプリにパーミッション(許可)を追加する。
3.VideoPlayerControllerを作成し、初期設定します。
4.動画再生プレイヤーを表示する。
5.動画を再生・一時停止する。
1.video_playerのDependencyを追加する。
dependencies:
flutter:
sdk: flutter
video_player:
2.アプリにパーミッション(許可)を追加する。
Android
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<application ...>
</application>
<uses-permission android:name="android.permission.INTERNET"/>
</manifest>
iOS
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
</dict>
3.VideoPlayerControllerを作成し、初期設定する
VideoPlayerControllerを作り、初期化する方法は以下のようになります。
1.StatefulWidgetを作成して、State Classを設定します。
2.State classに変数を追加し、VideoPlayerControllerを記憶させます。
3.VideoPlayerController.initializeから返されたFutureを保持するために、State classに変数を追加します。
4.initState methodでController作成し、初期化します。
5.dispose methodでControllerを削除します。
class VideoPlayerScreen extends StatefulWidget {
const VideoPlayerScreen({super.key});
@override
State<VideoPlayerScreen> createState() => _VideoPlayerScreenState();
}
class _VideoPlayerScreenState extends State<VideoPlayerScreen> {
late VideoPlayerController _controller;
late Future<void> _initializeVideoPlayerFuture;
@override
void initState() {
super.initState();
_controller = VideoPlayerController.network(
'動画.mp4',
);
_initializeVideoPlayerFuture = _controller.initialize();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
4.動画再生プレイヤーを表示する。
_initializeVideoPlayerFuture() が完了した後にWidgetを表示します。Controllerの初期化が終了するまで、FutureBuilder を使用してローディングスピナーを表示します。
FutureBuilder(
future: _initializeVideoPlayerFuture,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.done) {
return AspectRatio(
aspectRatio: _controller.value.aspectRatio,
child: VideoPlayer(_controller),
);
} else {
return const Center(
child: CircularProgressIndicator(),
);
}
},
)
5.動画を再生・一時停止する
初期状態では、動画は一時停止状態で開始されます。再生を開始するには、VideoPlayerControllerが持っているplay() という関数を呼び出します。一時停止したい場合は、pauseを呼び出します。
FloatingActionButton(
onPressed: () {
setState(() {
if (_controller.value.isPlaying) {
_controller.pause();
} else {
_controller.play();
}
});
},
child: Icon(
_controller.value.isPlaying ? Icons.pause : Icons.play_arrow,
),
)
参考 : Flutter.dev
金曜担当 – Ami
asahi at 2023年04月21日 10:00:00
Laravel Debugbar Package
Today, I would like to share about a package named laravel-debugbar.
Debugging is an essential part of the software development process, and Laravel provides a convenient package to make it easier. Laravel Debugbar is a powerful package that provides a debugging toolbar for your Laravel applications. It provides detailed information about the application’s performance, SQL queries, and more. In this blog, we will explore how to install Laravel Debugbar and use it to debug your Laravel applications.
Installation
Laravel Debugbar can be easily installed via Composer. Open up your terminal and navigate to your Laravel project directory. Then, run the following command:
composer require barryvdh/laravel-debugbar --dev
This will install the package and add it to your dev dependencies.
Next, you will need to add the service provider and alias in your config/app.php file. In the providers array, add the following:
Barryvdh\Debugbar\ServiceProvider::class,
And in the aliases array, add the following:
'Debugbar' => Barryvdh\Debugbar\Facade::class,
Once you have added the service provider and alias, you can publish the package assets by running the following command:
php artisan vendor:publish --provider="Barryvdh\Debugbar\ServiceProvider"
This will publish the package’s configuration file, views, and assets.
Usage
To start using Laravel Debugbar, you can simply add the following line of code to the beginning of your routes or controllers:
Debugbar::enable();
This will enable the debugbar for that specific route or controller.
To test that the debugbar is working correctly, you can add a simple query to your controller and view the results in the debugbar. Here is an example:
public function index()
{
$users = DB::table('users')->get();
return view('users', compact('users'));
}
You can loop through the $users variable and display the results. Then, open up your browser and navigate to the route that displays the users. You should see the debugbar at the bottom of the page, displaying information about the SQL query that was executed to retrieve the users.
Conclusion
Laravel Debugbar is a powerful package that makes it easy to debug your Laravel applications. It provides detailed information about the application’s performance, SQL queries, and more. In this blog, I have explored how to install Laravel Debugbar and use it to debug your Laravel applications. To learn more about Laravel Debugbar, check out the official documentation and GitHub repository:
GitHub URL: https://github.com/barryvdh/laravel-debugbar
Official Documentation: https://github.com/barryvdh/laravel-debugbar/blob/master/readme.md
This is all for now. Hope you enjoy that.
By Asahi
waithaw at 2023年04月18日 10:00:00
- 2023年04月14日
- 技術情報
Flutter 3.7リリースAndroidでフレーバーを利用する
Androidでのflavorの設定は、プロジェクトのbuild.gradleファイルで行うことができます。
Flutterプロジェクト内で、android/app/build.gradleにナビゲートします。
追加した商品フレーバーをグループ化するためにflavorDimensionを作成 します。Gradleは同一次元を共有するプロダクトフレーバーを結合することはありません。
dimension、resValue、applicationIdまたはapplicationIdSuffixの値と一緒に、希望のフレーバーでproductFlavorsオブジェクトを加えていきます。
各ビルドのアプリケーション名は、resValueに位置します。
applicationIdの代わりにapplicationIdSuffixを指定した場合は、「ベース」のアプリケーションIDに付加さ れます。
flavorDimensions "default"
productFlavors {
free {
dimension "default"
resValue "string", "app_name", "free flavor example"
applicationIdSuffix ".free"
}
}
参考 : Flutter.dev
金曜担当 – Ami
asahi at 2023年04月14日 10:00:00