Converting images into a pdf in python
- 2021年12月14日
- 技術情報
Last weekend, my friend asked me to help him something about rearranging images and converting them into a pdf with only portrait view and sorting by modified date . But the images were not sorted by that and some in landscape. They were in chaos. Actually there are many online tools to do that. But most of them were not compatible with all what I wanted. Then I decided to do with a python program and wrote the following small code block. Let’s take a look.
I’ve used the image library called Pillow(PIL) and built-in module named os.
Overall program flow is as follow.
- Request user inputs for a pdf filename with path and image folder path to be converted.
- With os module, image files are sorted by modified date.
- Looping the sorted files, rotate the images which are in landscape, to be in portrait with the help of Pillow(PIL) and push the images into an empty list named img_list[ ].
- Finally convert the images list to a pdf.
from PIL import Image
import os
# Function to sort by modified dates
def getfiles(dirpath):
a = [s for s in os.listdir(dirpath)
if os.path.isfile(os.path.join(dirpath, s))]
a.sort(key=lambda s: os.path.getmtime(os.path.join(dirpath, s)))
return a
# declare an empty list
img_list = []
# Request user input for pdf filename and image folder path
pdf_filename = input("Enter the filename of pdfincluding path to be exported : ")
folder = input("Enter the path of images folder : ")
files = getfiles(folder) # get the files sorted by modified dates
print('Processing....')
for count, filename in enumerate(files):
image = Image.open(folder+'/'+filename) #open each file
# To change as portrait layout for landscape images
width, height = image.size
if(width>height):
image = image.transpose(Image.ROTATE_90)
# Append each processed image in img_list[]
img_list.append(image)
# All images in img_list[] are converted to a pdf
img_list[0].save(pdf_filename, "PDF" ,resolution=100.0, save_all=True, append_images=img_list[1:])
print("Done!")
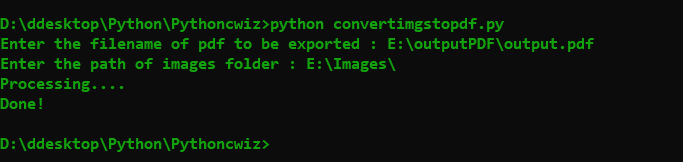
This program is very simple but it can be modified to be more useful for other cases. I hope you enjoy that.
By Asahi
waithaw at 2021年12月14日 10:00:00